This post goes over some common git commands and explains what they do.
Stages and File states
Git tracks changes to files in snapshots and stores these in its database. Files managed through git can have one of three states and go through three stages in the git workflow.
File States
Files have the following states:
Stage | Explanation |
Modified | A file that git knows about has been changed in some way but the change hasn’t been committed. |
Staging | The changed file is marked to go into the next commit. File tagged to go into next commit using git add . Adds changes to staging area. |
Committed | The change or modifications to the file are stored in the git database. |
Git sections
Every Git project has these three sections:
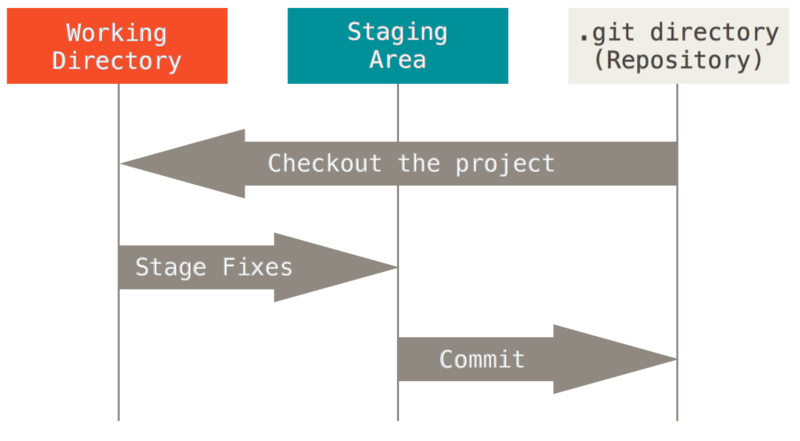
Section | Description |
Working tree | Single checkout of a version of the project |
Staging Area | A file that stores information about what will go into the next commit. These are files git knows about. |
Git directory | This is where git stores metadata about your project and its object database. This is the .git folder |
The basic workflow in git is you make changes to files in the working tree, stage the ones you want to go into the next commit, and then do a commit. The commit stores a snapshot of the changes permanently to your Git directory.
Basic Commands
Here’s a list of a few commands you are likely to use to manage a local git project.
Command | Description |
git init | Creates a .git subdirectory and initialises a new git project |
git clone <URL> | Pull down every version and history of every file in the linked project. Initialises .git subdirectory and checks out a copy of the latest version of the project |
git add <file> | Tells git about a file. This tracks and stages the file. |
git status | Shows the status or the state of files. Use the -s flag for more concise output. |
git diff | Shows you what has changed between two commits |
git diff --staged | Shows you what has changed in staged files (i.e files that have been git add -ed) |
git diff --cached | Same as git diff --cached , it shows changes to staged files |
git commit | Commits or saves changes in staged files to the git database. |
git commit -v | Shows what you changed in the commit message |
git commit -a -m <msg> | Skips staging area and commits all modified files. |
git rm <file> | Delete a file and remove it from the staging area or index. |
git rm -f | Force git to remove a modified file |
git rm --cached <filename> | Make git forget about a file without deleting it from the filesystem. |
git mv <file_from> <file_to> | Move or rename a file; equivalent to:mv file_from file_to git rm file_from git add file_to |
git log | View commit history. This command takes many different options that affect its output. I will put up a separate post about it. |
git commit --amend | Edit the previous commit. Useful for removing files or changing commit message. |
git reset HEAD <file> | Reset a file back to being unstaged modified. |
git checkout -- <file> | Unmodify a modified file |
git restore --staged <file> | Unstage a file |
git restore <file> | Unmodify a modified file back to the state it was in in the last commit. |
git remote | A remote is a version of the project you have hosted elsewhere. git remote shows the server you cloned from, usually called origin. |
git remote -v | Includes URLs of the remotes associated with your project. |
git remote add <name> <URL> | Add a new remote. Use git fetch <name> to fetch from the remote. |
git push <remote_name> <branch> | Push changes to a remote. For example, you can do git push origin master to push to the master branch on the origin remote. |
git remote show <name> | Show information about the remote. |
git remote rename | Rename a remote. Example: git remote rename pb vuyisile renames the pb remote to vuyisile |
git remote remove <name> | Remove the named remote. |